There are a couple of scenario's when it comes to protecting applications using the Azure Active Directory (See here):
These are the five primary application scenarios supported by Azure AD:
- Web Browser to Web Application: A user needs to sign in to a web application that is secured by Azure AD.
- Single Page Application (SPA): A user needs to sign in to a single page application that is secured by Azure AD.
- Native Application to Web API: A native application that runs on a phone, tablet, or PC needs to authenticate a user to get resources from a web API that is secured by Azure AD.
- Web Application to Web API: A web application needs to get resources from a web API secured by Azure AD.
- Daemon or Server Application to Web API: A daemon application or a server application with no web user interface needs to get resources from a web API secured by Azure AD.
You mention you have registered a Native Application. I assume you need to authenticate against the Azure Active Directory (AAD from now on) to gain access to a protected web api or web app (scenario #3) so you have to register that one as well.
static void Main(string[] args)
{
GetTokenAsync().Wait();
}
static async Task<string> GetTokenAsync()
{
string Tenant = "mytest.onmicrosoft.com";
string Authority = "https://login.microsoftonline.com/" + Tenant;
string GatewayLoginUrl = "https://login.microsoftonline.com/something/wsfed";
string ClientId = "something";
Uri RedirectUri = new Uri("http://something");
AuthenticationContext context = new AuthenticationContext(Authority);
PlatformParameters platformParams = new PlatformParameters(PromptBehavior.Auto, null);
AuthenticationResult result = await context.AcquireTokenAsync(GatewayLoginUrl, ClientId, RedirectUri, platformParams);
return result.ToString();
}
Tenant
is the name of the AAD domain, it seems you got that one right
Authority
is "https://login.microsoftonline.com/" + Tenant
, so it seems you got that one right too
GatewayLoginUrl
is the App Id Uri of the application that you are protecting
ClientId
is the Application Id of the native application
RedirectUri
is the Redirect Uri of the native application
Application to Protect:
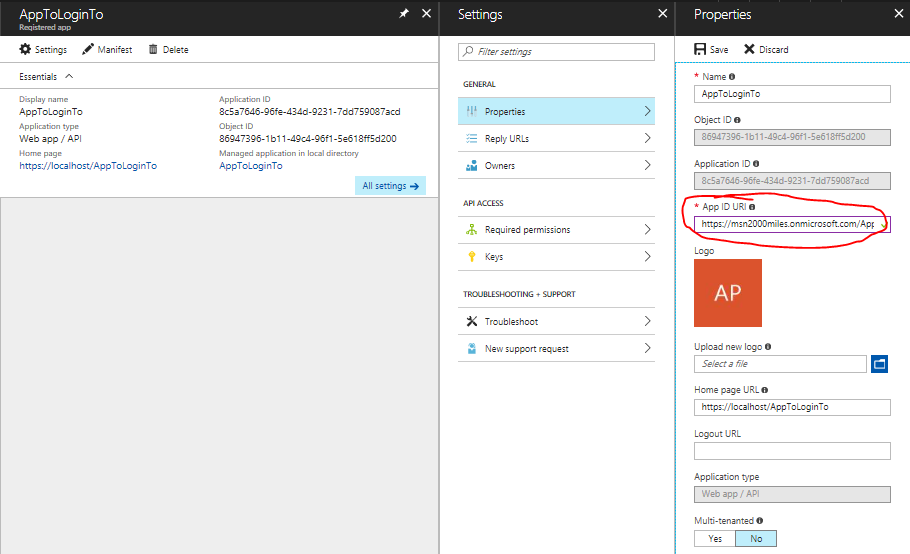
You get the GatewayLoginUrl
from here.
Native Application that accesses the Application to Protect:

You get the ClientId
and RedirectUri
from here.
Other references
You can see a full walkthrough for a native application here
For a global overview of accessing AAD protected applications using a native app see the docs